- Published on
A Comprehensive Guide to master XPath Queries in Java
- Authors
- Name
- Khalil
- @Im_Khalil
Recently I came across a use case which involves retrieving the ID of a specific node in an XML document based on the ID of its child node. Initially, I considered parsing the document using regular expressions, but I realized that this approach would be costly in terms of performance. So, I decided to explore alternative solutions and discovered that XPath operations are faster and more efficient for obtaining the required information in such scenarios. I dedicated some time to learning how to write and execute XPath queries.
After about an hour of research and experimentation, I successfully wrote a query that provides the desired result. Here is the XPath query I came up with to retrieve the ID of the ancestor node:
//p[@id='someid']/ancestor::bookNode[1]/@id)[1]
To ensure the correctness of my XPath expression, I used a helpful website that allows you to input a sample XML string and the XPath expression to evaluate it.
https://www.freeformatter.com/xpath-tester.html#before-output
The following guide will help you to use the xpath queries in java code.
What is XPath?
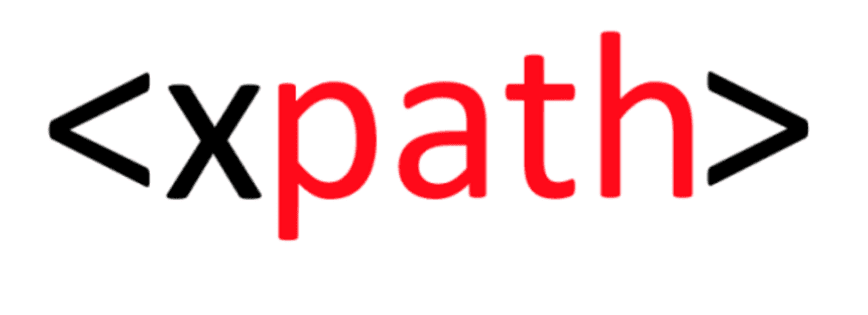
Setting up the Environment:
Before we start writing XPath queries in Java, let's make sure we have the necessary tools and libraries set up. You'll need the following:
Java Development Kit (JDK):
Make sure you have the latest version of JDK installed on your system.
An Integrated Development Environment (IDE):
Choose an IDE of your preference, such as Eclipse, IntelliJ IDEA, or NetBeans. These IDEs provide excellent support for Java development and make it easier to write and test XPath queries.
Libraries:
You'll need the XPath library to perform XPath operations in Java. The most commonly used library is the Java API for XML Processing (JAXP), which is part of the Java SE platform. It provides built-in support for XPath queries through the javax.xml.xpath package.
Writing XPath Queries in Java:
To write XPath queries in Java, you need to follow these steps:
Step 1: Create an XPath object:
Instantiate an XPath object using the XPathFactory class, which is part of the JAXP library.
import javax.xml.xpath.*;
XPathFactory xpathFactory = XPathFactory.newInstance();
XPath xpath = xpathFactory.newXPath();
Step 2: Compile the XPath expression:
Compile the XPath expression using the compile() method of the XPath object.
XPathExpression expression = xpath.compile("<XPath expression>");
Step 3: Evaluate the XPath expression:
Evaluate the compiled XPath expression on the XML or HTML document using the evaluate() method.
Object result = expression.evaluate(document, <resultType>);
Here, the document represents the XML or HTML document, and <resultType>
specifies the type of result you expect, such as NODESET, STRING, NUMBER, or BOOLEAN.
Step 4: Process the result:
Process the result returned by the evaluate() method based on its type. For example, if the result type is NODESET, you can iterate over the nodes and extract the required data.
NodeList nodes = (NodeList) result;
for (int i = 0; i < nodes.getLength(); i++) {
Node node = nodes.item(i);
// Process the node as needed
}
XPath Query Examples:
Let's explore a few common examples of XPath queries in Java:
- Selecting nodes by element name:
XPathExpression expression = xpath.compile("//book");
NodeList books = (NodeList) expression.evaluate(document, XPathConstants.NODESET);
- Selecting nodes by attribute value:
XPathExpression expression = xpath.compile("//book[@category='fiction']");
NodeList fictionBooks = (NodeList) expression.evaluate(document, XPathConstants.NODESET);
- Selecting nodes by text content:
XPathExpression expression = xpath.compile("//book[contains(text(),'Java')]");
NodeList javaBooks = (NodeList) expression.evaluate(document, XPathConstants.NODESET);
Bonus Information : Xpath cheatsheet